Python is a powerful programming language used by data scientists and developers to extract, manipulate, and analyze data. It provides several libraries and modules for data handling, including the CSV module. The CSV (Comma Separated Values) module allows the data to be stored in tabular form and is widely used for exchanging data between different applications. In this article, we will explore reading CSV files in Python.
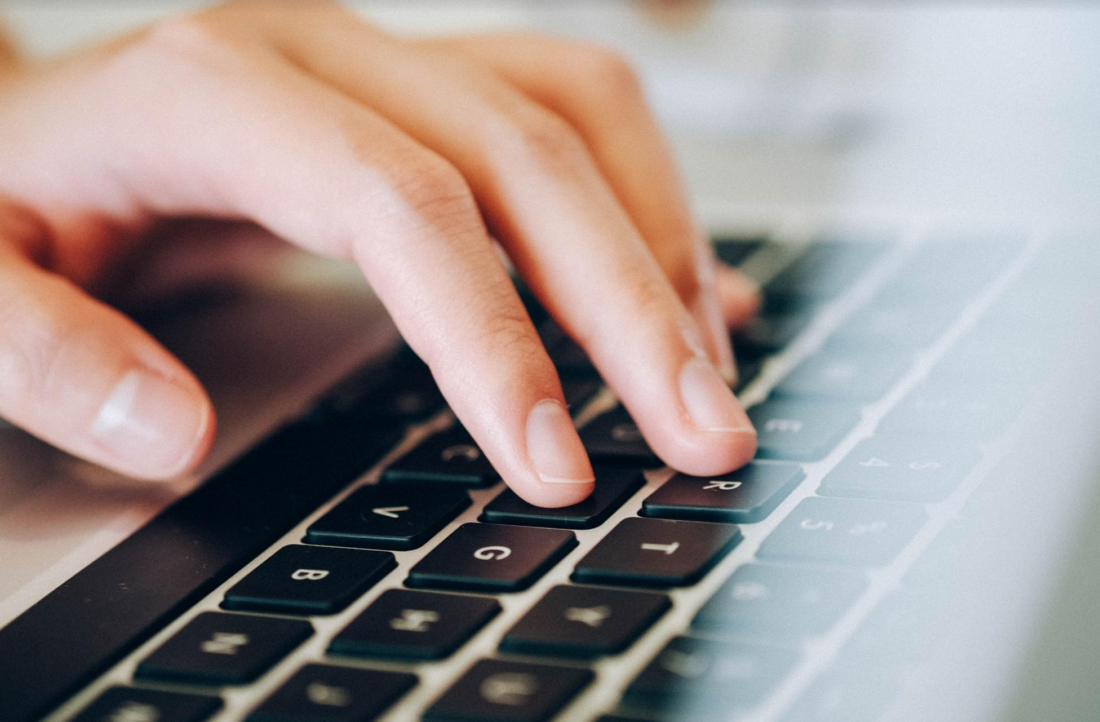
Step 1: Import the Required Libraries
Before exporting the data to a CSV file, we need to import the necessary libraries. The CSV library is already included in Python’s standard library, so we can directly import it. Additionally, we may need to import other libraries like Pandas, which provide advanced data manipulation features.
“`
import csv
import pandas as pd
“`
Step 2: Define the Data
Next, we must define the data we want to export to a CSV file. For this tutorial, we will create a simple dictionary containing some sample data.
“`
data = {‘Name’: [‘John’, ‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 27, 22],
‘City’: [‘New York’, ‘Paris’, ‘London’, ‘Tokyo’]}
“`
Step 3: Write the Data to a CSV File
Now that we have defined the data, we can write it to a CSV file using the csv module’s writerow() function. We will create a new file called ‘data.csv’ and write the data to it.
“`
with open(‘data.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file)
writer.writerow([‘Name’, ‘Age’, ‘City’])
for i in range(len(data[‘Name’])):
writer.writerow([data[‘Name’][i], data[‘Age’][i], data[‘City’][i]])
“`
In the above code, we have opened a file named ‘data.csv’ in write mode and created a writer object. We have also written the header row containing the column names. Finally, we have looped through the data dictionary and written each row to the CSV file.
Step 4: Export Data Using Pandas
In addition to the CSV module, Pandas provides a powerful and convenient way to export data to a CSV file. We can use the to_csv() function provided by the Pandas module to export data to a CSV file.
“`
df = pd.DataFrame(data)
df.to_csv(‘data.csv’, index=False)
“`
In the above code, we have created a DataFrame using the data dictionary and used the to_csv() function to export the data to a CSV file. The index=False parameter is used to exclude the index column from the CSV file.
In this article, we have explored how to export data to CSV files in Python using the Cule and Pandas modules. The CSV module provides a straightforward way to write data to a CSV file, while the Pandas module provides advanced data manipulation features and is more convenient for working with large datasets. With these tools, exporting data to a CSV file in Python is quick and easy.